Unix tools
The GNU Compiler Collection
A compiler is a program that translates high-level language instructions
into machine code. GCC, the GNU Compiler Collection, can translate
C (gcc
), C++ (g++
), Objective-C, Objective-C++, Fortran
(gfortran
), Ada, and Go (gccgo
). It also provides code
libraries for these languages.
The C programming language was developed by Dennis Ritchie at Bell Labs in 1972. Object-oriented features were added by Bjarne Stroustrup in 1979 under the name C++ (computing humor: ++ is C’s increment operator). Since then, C++ has undergone many refinements, and it has become the most common language for application development.
See also
$ cat > hello.cpp
#include <iostream>
using std::cout;
using std::endl;
int main()
{
cout << "Hello World!" << endl;
return 0;
}
[ctrl-d]
$ g++ -o hello hello.cpp
$ ls -F
hello* hello.cpp
$ ./hello
Hello World!
Let us work through the program hello.cpp
step by step.
Lines that begin with the hash symbol (#
) are not part of
the C++ language; rather, they are preprocessor directives.
In this case, #include
tells the compiler to read in the
header file iostream
, which contains definitions related to input and output.
C++ supports a feature called namespaces. Objects and functions
provided as part of the standard library live in the std
namespace.
The statement using std::
name indicates that name should be
added to the common namespace. (We could have left out the using
std::cout
declaration and simply refered to std::cout
throughout
the program.) cout
is a handle to the Unix stdout stream. The
desired output—here, the character string "Hello World!"
and the
line feed endl
—are chained together using the <<
operator.
Every C++ program must contain one function called main
. This is the
first and only function executed. Once it completes, it gives control
back to the operating system and returns an integer, with zero
indicating normal operation and other integers denoting various error
states.
g++
invokes the gcc C++ compiler, which processes the file
hello.cpp
. The option -o hello
indicates that the compiler
should produce an executable file named hello
. If the -o
option
is not included, the executable is named a.out
by default.
Make files
The make
program provides a mechanism for automating the compilation
of your programs. It supports user-defined rules and can track changes
to your code based on the date stamp of the files you declare as
dependencies.
See also
$ cat > makefile
hello: hello.cpp
g++ -o hello hello.cpp
[ctrl-d]
$ make
make: `hello' is up to date.
$ touch hello.cpp
$ make
g++ -o hello hello.cpp
$ ./hello
Hello World!
$ cat > makefile
CC=g++
CFLAGS=-O2 -ansi -pedantic
hello: hello.cpp
.cpp:
$(CC) -o $@ $@.cpp $(CFLAGS)
clean:
rm hello 2> /dev/null
[ctrl-d]
$ make
$ touch hello.cpp
$ make
make: `hello' is up to date.
$ make clean
rm hello 2> /dev/null
$ make
g++ -o hello hello.cpp -O2 -ansi -pedantic
$ ./hello
Hello World!
Vi and Vim
The cat >
trick is a quick and dirty way to enter text into file,
but in general you will want to use a more full-featured text editor
when writing code. vi
is the historical, default Unix line editor.
vim
(Vi IMproved) and nvim
(NeoVim) are modern rewrites of the
classic vi
that provide syntactic colour highlighting and other
goodies.
See also
The behavior of Vi-style editors is modal and rather different than
what you may be used to. Try launching vi
and entering exactly the
keystrokes shown below (where <cr> is the carriage return or enter key
and <esc> is the escape key). Then check out the help system to learn
more.
$ vi course.rst
iIntroduction<esc>yypVr=A<cr><cr>scientific computing<esc>?sci<cr>~w~IPhys 540: <esc>:qw
$ cat course.rst
Introduction
============
Phys 540: Scientific Computing
$vi
:help
Gnuplot
Gnuplot provides both an interactive plotting environment and a scripting system for producing data plots. The output can be sent to the screen or to disk in a variety of graphics formats.
See also
- Online references:
Here is an example of gnuplot run as a script.
$ whereis gnuplot
/bin/gnuplot
$ cat > linear.dat
1 3.1 0.3
2 4.9 0.5
3 6.95 0.4
4 9.2 0.7
[ctrl-d]
$ cat > plot.gp
#!/bin/gnuplot -persist
f(x) = f0 + f1*x
fit f(x) "linear.dat" using 1:2:3 via f0,f1
plot[0:5] "linear.dat" using 1:2:3 with errorbars, f(x)
[ctrl-d]
$ gnuplot -persist plot.gp
$ chmod +x plot.gp
$ ./plot.gp
The using
specification determines which columns in the data file
are plotted. In the examples above, using 1:2:3 with errorbars
plots
column 2 (y-axis) versus column 1 (x-axis) with column 3 acting as the
error on each point. It is also possible to perform mathematical
transformations on the data. Such transformations are enclosed in
parentheses and a dollar sign precedes the column number: e.g., using
(1.0/$1):($2+0.5*$3)
would plot the value in column 2 plus half the
value in column 3 versus the inverse of the value in column 1.
Here is an example of an interactive session.
$ gnuplot
gnuplot> set terminal pdfcairo
Terminal type is now 'pdfcairo'
gnuplot> set output 'linear.pdf'
gnuplot> plot[0:5][0:10] "linear.dat" using 1:2 with lines
gnuplot> unset output
gnuplot> set terminal qt
gnuplot> replot
gnuplot> quit
$ ls
linear.dat linear.pdf
$ evince linear.pdf &
Asymptote
if(!settings.multipleView) settings.batchView=false;
settings.tex="pdflatex";
defaultfilename="tangent";
if(settings.render < 0) settings.render=4;
settings.outformat="";
settings.inlineimage=true;
settings.embed=true;
settings.toolbar=false;
viewportmargin=(2,2);
//usepackage("stix");
usepackage("newtxtext");
usepackage("newtxmath");
import graph;
size(8cm,6cm,IgnoreAspect);
// x(t) = 3t^3 - 2t^2 + 5t - 10 = ((3t - 2)t + 5)t - 10
real x(real t) {return ((3*t - 2)*t + 5)*t - 10; }
pair X(real t) {return (t,x(t));}
// x'(t) = 9t^2 - 4t + 5 = (9t - 4)t + 5
real xp(real t) {return (9*t - 4)*t + 5 ;}
real t1 = 0.5;
real t2 = 2;
real f(real t) { return x(t2) + xp(t2)*(t-t2); }
real[] horiz={t1,t2};
real[] vert={x(t1),x(t2)};
draw(graph(horiz,vert),rgb(0.2,0.55,0.2)+linewidth(1));
label("$t_1$",X(t1),NW);
draw(graph(f,t2-0.75,t2+0.75,operator ..),rgb(0.55,0.2,0.2)+linewidth(1));
label("$t_2$",X(t2),SE);
draw(graph(x,-1,3,operator ..),rgb(0.2,0.2,0.55)+linewidth(1.2));
dot(X(t1),linewidth(3.5));
dot(X(t2),linewidth(3.5));
xaxis("$t$ (s)",BottomTop,LeftTicks);
yaxis("$x(t)$ (m)",LeftRight,RightTicks(trailingzero));
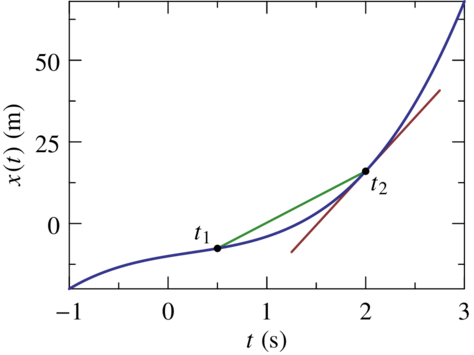
GhostScript
PostScript is a page description language from Adobe. It is the native language of most laser printers and is still the most common way to encode printed graphics. In contrast with the Portable Document Format (PDF), which is derived from PostScript, PostScript is a true programming language.
See also
A PostScript file always begins with the two-character identifier %!
and
ends with the showpage
command. PostScript files can be
viewed using GhostView (gv
), which is a graphical front end for
GhostScript, the GNU PostScript interpreter.
$ cat > figure.ps
%!
newpath
100 200 moveto
200 250 lineto
100 300 lineto
2 setlinewidth
stroke
newpath
300 300 75 0 180 arc
closepath
gsave
0.5 setgray
fill
grestore
4 setlinewidth
1 0 0 setrgbcolor
stroke
showpage
[ctrl-d]
$ gv figure.ps &
If you are using Linux with the GNOME desktop, you may prefer evince
to gv
. Under macOS, the open
command will send PostScript files
to Preview.app
for viewing.
Encapsulated PostScript (EPS) is a restricted version of PostScript that
was designed to make it easier to embed PostScript graphics in other
applications. There are three rules to follows. The file should consist
of just one page. The first line of the file should be %!PS-Adobe-3.0
EPSF-3.0
. There must be a correctly formed BoundingBox comment, of
the form %%BoundingBox:
xmin ymin xmax ymax, which tells the
application that embeds the EPS file how large the image is.
$ echo %\!PS-Adobe-3.0 EPSF-3.0 > figure.eps
$ echo %%BoundingBox: 50 150 400 400 >> figure.eps
$ cat figure.ps | sed 's/showpage//' | sed 's/%\!//' >> figure.eps
$ more figure.eps
%!PS-Adobe-3.0 EPSF-3.0
%%BoundingBox: 50 150 400 400
newpath
100 200 moveto
200 250 lineto
100 300 lineto
2 setlinewidth
stroke
newpath
300 300 75 0 180 arc
closepath
gsave
0.5 setgray
fill
grestore
4 setlinewidth
1 0 0 setrgbcolor
stroke
[ctrl-d]
$ gv figure.eps &
ImageMagick
Process images or transform between file formats using the magick
command.
See also
$ magick convert -density 300 tangent.pdf -resize 50% tangent.png
LaTeX
LaTeX is a document markup language built on top of the TeX typesetting system. It is used by scientists and mathematicians to lay out documents that combine text, mathematics, and figures.
See also
\documentclass{article}
%\usepackage[top=1.0in , bottom=1.0in, left=1.0in, right=1.0in]{geometry}
\usepackage{amsmath,amssymb,graphicx}
%\usepackage{newtxtext,newtxmath}
\usepackage{stix}
\begin{document}
\title{Phys 540 --- Assignment 1}
\author{Student's name}
\date{Wednesday, July 1, 2020}
\maketitle
\section{\label{statement} Statement of the problem}
We are asked to compute the series
\begin{equation} \label{series}
\begin{split}
S_N &= \sum_{n=1}^N \frac{1}{n^2}\\
&= 1 + \frac{1}{4} + \frac{1}{9} + \cdots
\end{split}
\end{equation}
in the limit $N \to \infty$.
\section{Solution and implementation}
Here we show how to evaluate Eq.~\eqref{series}, which was presented in
Sect.~\ref{statement}.
% the percentage sign is a comment
% we are not including the encapsulated postscript figure
%\includegraphics{figure.eps}
% nor are we including this one in portable document format
%\includegraphics{figure.pdf}
\end{document}
The traditional way to compile produces a dvi file intermediary.
$ ls
assignment1.tex
$ latex assignment1.tex
$ latex assignment1.tex # run a second time to resolve references
$ ls
assignment1.aux assignment1.log
assignment1.dvi assignment1.tex
$ xdvi assignment1.dvi &
$ dvips -o assignment1.ps assignment1.dvi
$ gv assignment1.ps &
The more modern approach is to compile directly to a pdf.
$ ls
assignment1.tex
$ pdflatex assignment1.tex
$ pdflatex assignment1.tex
$ ls
assignment1.aux assignment1.pdf
assignment1.log assignment1.tex
$ evince assignment1.pdf
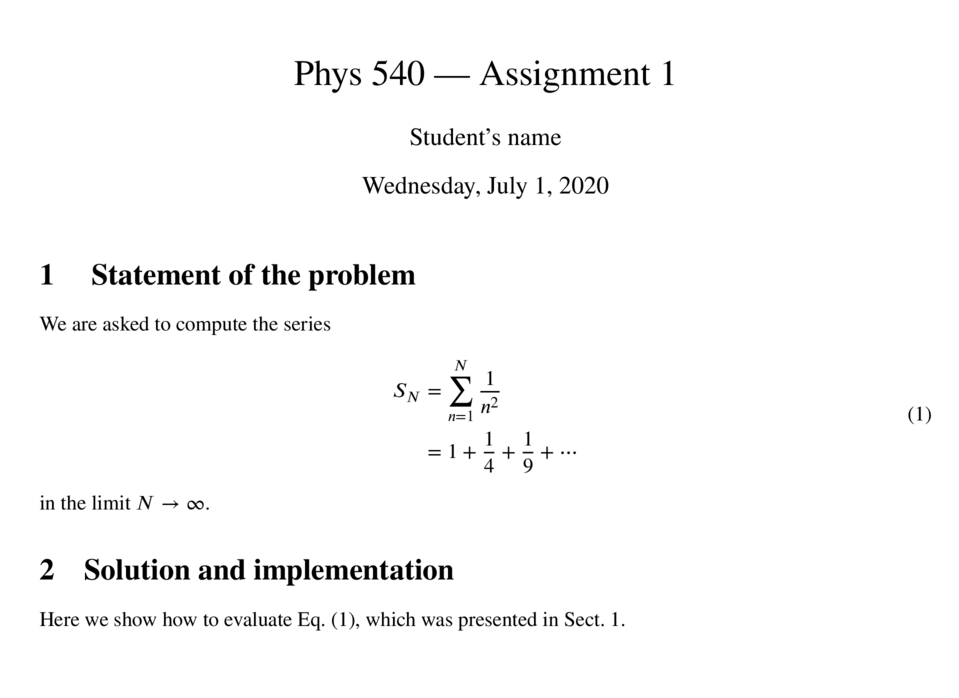
Archival and compression
The tar
(Tape ARchive) command dates back to the days when computer
memory was backed up to tape. Nonetheless, this command remains useful
as a tool for archiving directories. An archive is a single file that
encodes the many files and subdirectories that a directory may contain.
Archives can be created and extracted using tar cf
and tar xf
.
To reduce the file’s size, archives are commonly compressed using
gzip
to produce a so-called tarball, which carries a tar.gz
file extension (sometimes reduced to tgz
).
$ mkdir beach_1
$ touch beach_1/report.tex
$ touch beach_1/mycode.cpp
$ touch beach_1/output.dat
$ ls
beach_1/
$ ls beach_1
mycode.cpp output.cpp report.tex
$ tar cf beach_1.tar beach_1
$ ls
beach_1/ beach_1.tar
$ gzip beach_1.tar
$ ls
beach_1/ beach_1.tar.gz
$ rm beach_1/*
$ rmdir beach_1
$ ls
beach_1.tar.gz
$ gunzip beach_1.tar.gz
$ tar xf beach_1.tar
$ ls
beach_1/ beach_1.tar.gz
$ ls beach_1
mycode.cpp output.cpp report.tex
In this example, touch
simply creates an empty file with the given
filename. Remember that *
is a wildcard. The command rm
beach_1/*
instructs the computer to delete all files inside the
beach_1
directory.
Note that the gzip
/gunzip
steps can be performed automatically
by invoking tar
with the z
option
$ ls beach_1
mycode.cpp output.cpp report.tex
$ tar czf beach_1.tar.gz beach_1
$ ls
beach_1/ beach_1.tar.gz
$ rm beach_1/*
$ rmdir beach_1
$ ls
beach_1.tar.gz
$ tar xzf beach_1.tar
$ ls
beach_1/ beach_1.tar.gz
$ ls beach_1
mycode.cpp output.cpp report.tex